Migrate from an earlier version
This page details breaking changes from previous versions, so you understand the development effort required to update your app and take advantage of the latest features.
Versioning
We try to limit breaking or significant changes to major version increments. The three digits in our versioning scheme represent major, minor, and patch increments respectively.
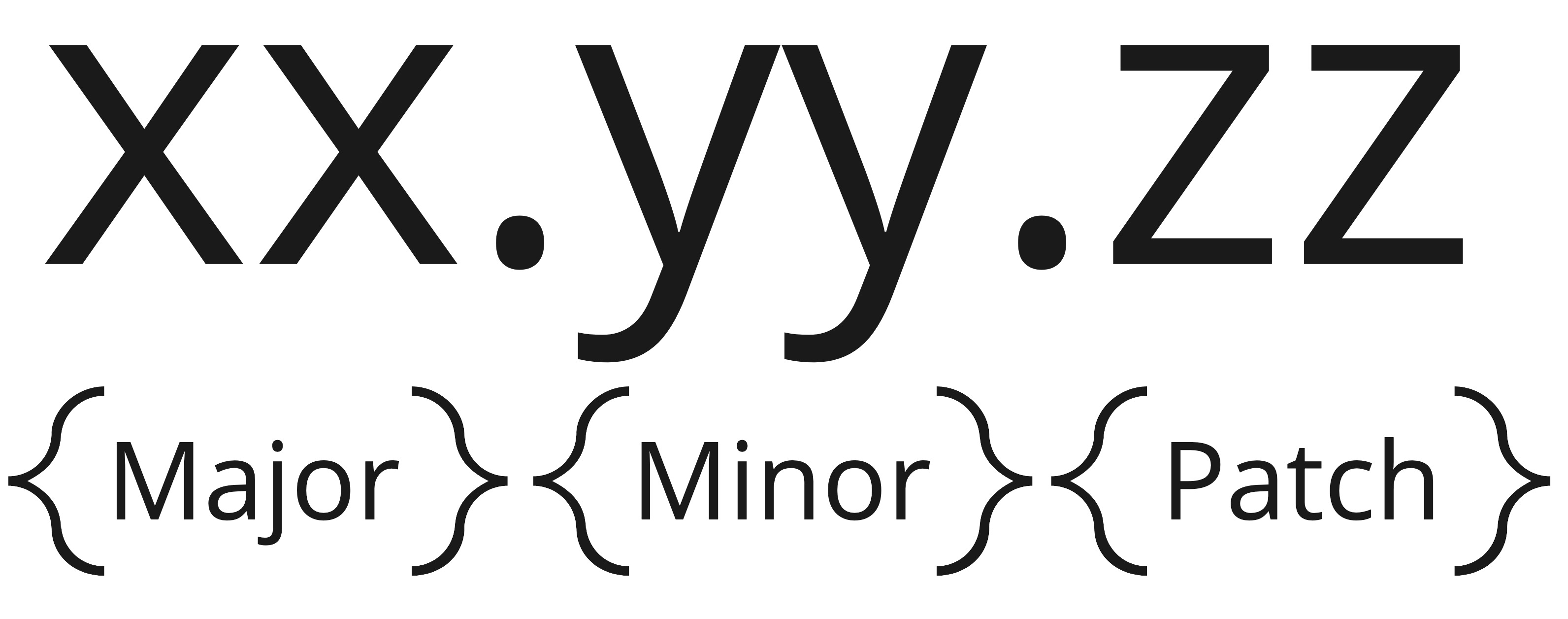
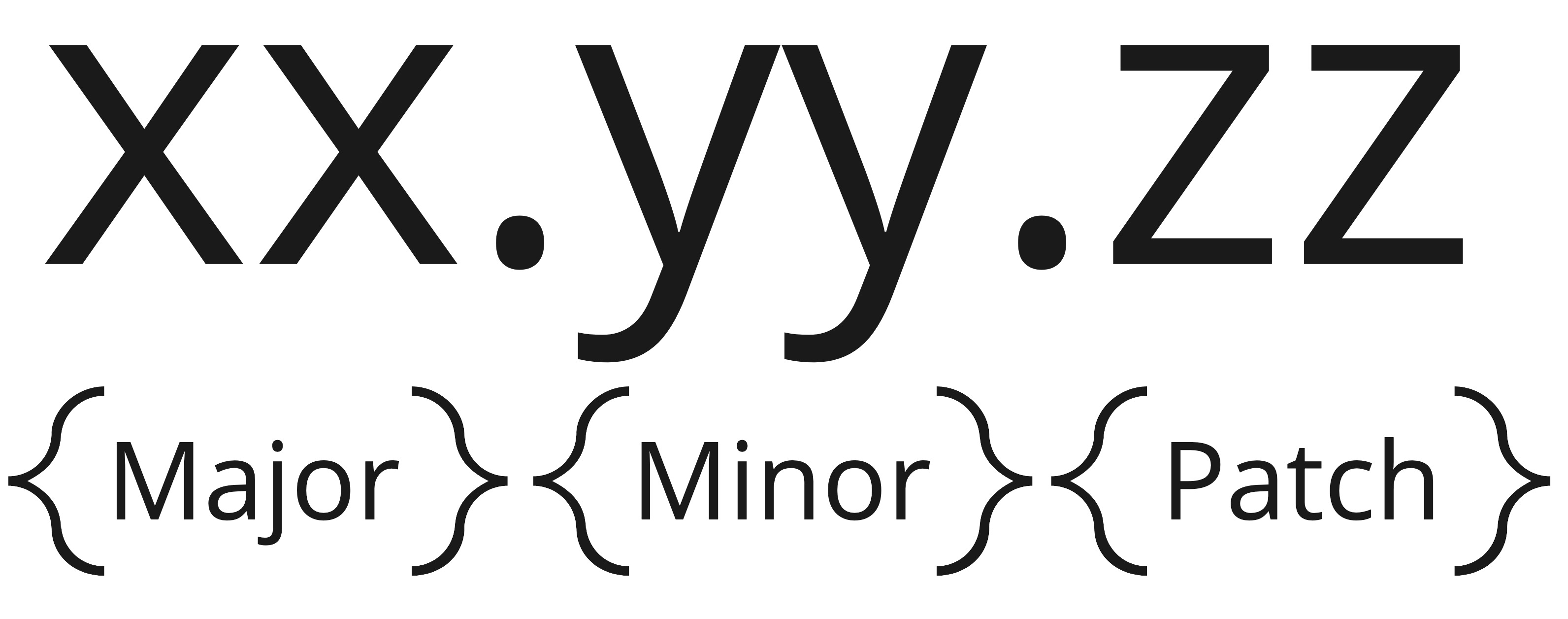
- Major: may include breaking changes, and generally introduces significant feature updates.
- Minor: may include new features and fixes, but won’t include breaking changes. You may still need to do some development to use new features in your app.
- Patch: Increments represent minor fixes that should not require development effort.
Upgrade from 2.x to 3.x
Installing and updating our React Native SDK got easier.
After you install the CustomerIO React Native SDK version 3.x, open your ios/Podfile
and follow all 5 steps shown in this code block below:
# 1. This line is required by the FCM SDK. If you encounter problems during 'pod install', add this line to your Podfile and try 'pod install' again.
use_frameworks! :linkage => :static
target 'YourApp' do # Note: 'YourApp' is unique to your app. This is here for example purposes, only.
# 2. Remove all 'pod CustomerIO...' lines (such as the example below).
pod 'CustomerIO/MessagingPushAPN', '~> 2' # Remove me
# 3. Add one of these new lines below:
# If you use APN for your push notifications on iOS, install the APN pod:
pod 'customerio-reactnative/apn', :path => '../node_modules/customerio-reactnative'
# If you use FCM for your push notifications on iOS, install the FCM pod:
pod 'customerio-reactnative/fcm', :path => '../node_modules/customerio-reactnative'
end
target 'NotificationServiceExtension' do
# 4. Remove all 'pod CustomerIO...' lines (such as the example below).
pod 'CustomerIO/MessagingPushAPN', '~> 2' # Remove me
pod 'FirebaseMessaging' # Remove me, unless you need to specify a specific version
pod 'Firebase' # Remove me, unless you need to specify a specific version.
# 5. Add one of these new lines below:
# ⚠️ Important: Notice these lines of code include "-richpush" in it making it unique to the host app target above.
# If you use APN for your push notifications on iOS, install the APN pod:
pod 'customerio-reactnative-richpush/apn', :path => '../node_modules/customerio-reactnative'
# If you use FCM for your push notifications on iOS, install the FCM pod:
pod 'customerio-reactnative-richpush/fcm', :path => '../node_modules/customerio-reactnative'
end
After you modify your Podfile
, run the command pod update --repo-update --project-directory=ios
to make your changes to ios/Podfile
go into effect.
Upgrade from 1.x to 2.x
Rich push initialization(iOS)
If you followed our docs to setup rich push in your app, you should have a Notification Service Extension
file in your code base.
Due to the behavior of Notification Service Extensions in iOS, you need to initialize the Customer.io SDK in your Notification Service Extension. In the case that you use Objective-C, you must add the code snippet below into the Swift handler file that you created in NotificationService Extension.
class NotificationService: UNNotificationServiceExtension {
override func didReceive(
_ request: UNNotificationRequest,
withContentHandler contentHandler: @escaping (UNNotificationContent) -> Void
) {
// Make sure to initialize the SDK at the top of this function.
CustomerIO.initialize(siteId: "YOUR SITE ID", apiKey: "YOUR API KEY", region: Region.US) { config in
config.autoTrackPushEvents = true
}
...
}
}
See our docs for rich push to learn more about rich push setup, SDK initialization, and SDK configuration.
Firebase users must manually install Firebase dependencies
We removed all Firebase SDKs as dependencies from the CustomerIO/MessagingPushFCM
Cocoapod. If you send messages to your iOS app using FCM, you’ll need to install the Firebase Cloud Messaging (FCM) dependencies in your Podfile
on your own.
pod 'Firebase'
pod 'FirebaseMessaging'
We fixed a bug in our iOS modules that may impact your data
SDK functions that let you send custom data—trackEvent
, screen
, identify
and deviceAttribute
calls—may have been impacted by a bug in our iOS v1 modules that converted keys in your custom data to snake_case
. This bug is fixed in v2 of the SDK. You will see your data in Customer.io exactly as you pass it to the SDK.
This bug didn’t surface with all data; it did not affect you if you already snake-cased your data; and it did not affect your Android users..
// If you passed in custom attributes using camelCase keys:
data = {"firstName": "Dana"}
// The SDK v1 may have converted this data into:
data = {"first_name": "Dana"}
// Or, if you used a different format that was not snake_case:
data = {"FIRSTNAME": "Dana"}
// The SDK v1 may have converted this data into:
data = {"f_irstname": "Dana"}
You don’t need to do anything before you update. But we strongly recommend that you go to Data & Integrations > Data Index and audit your attributes and events to determine if the v1 SDK reshaped your data. Make sure that updating to the 2.x SDK won’t impact your segments, campaigns, etc by sending data in a different (but expected) format to Customer.io.
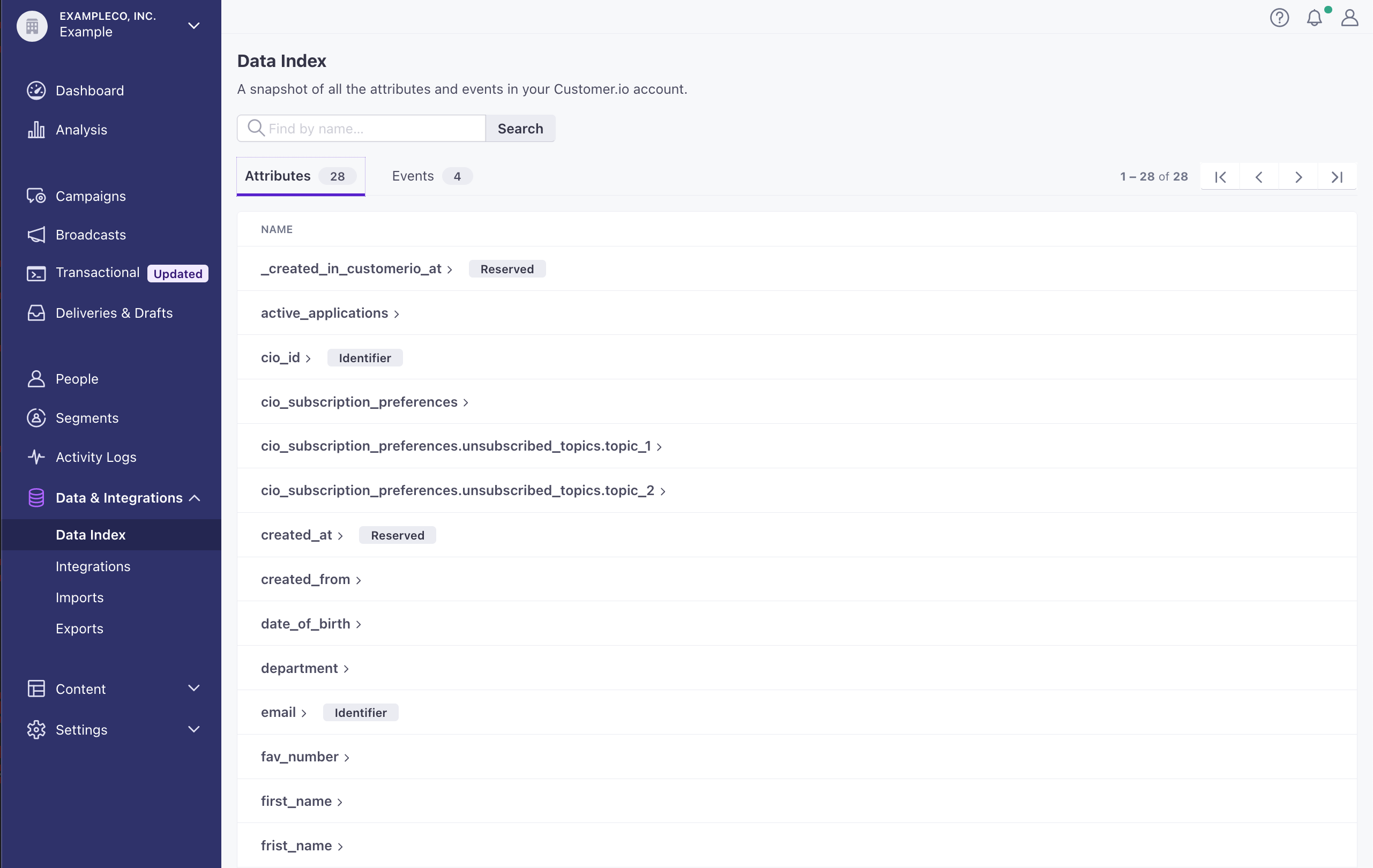
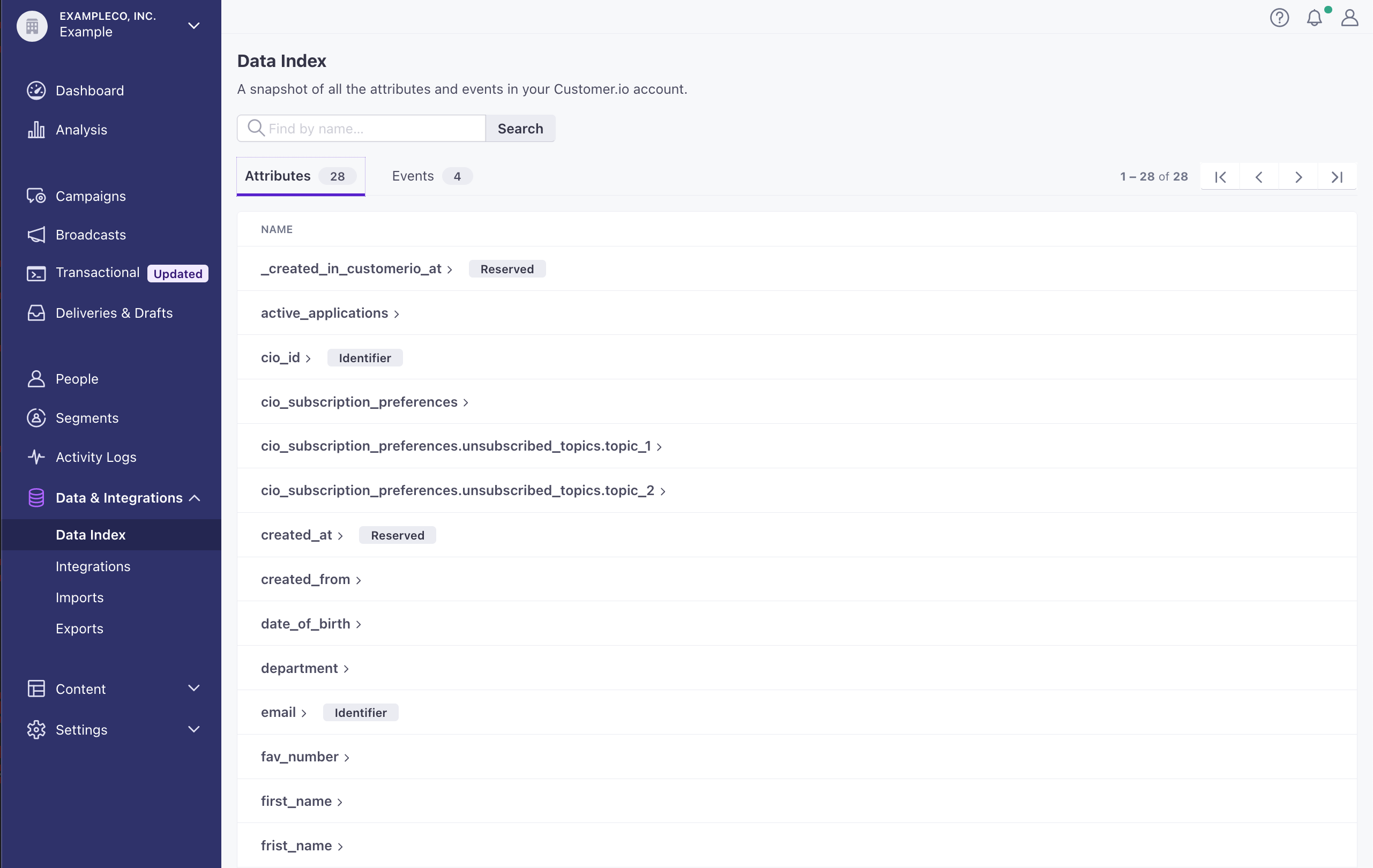
If your data was affected, you can either:
- (Recommended) Update your attributes, segments, and other information stored in Customer.io to use your original data format.
- Set your app to continue using the snake-cased data passed by the 1.x SDK.
Option 1 (Recommended): Update your data in Customer.io
For Events: trackEvent
and screen
calls
Unfortunately, you can’t modify past events sent by trackEvent
or screen
calls. But, before you move forward with the 2.0 SDK, you can can update your segments, campaigns, and other Customer.io assets to use your original, not-reshaped data format.
For segmentsA group of people who match a series of conditions. People enter and exit the segment automatically when they match or stop matching conditions., you should use OR conditions with the bugged, snake-cased format and your preferred data format. This ensures that people enter your segments and campaigns whether they use your app with the 1.x or 2.x SDKs.
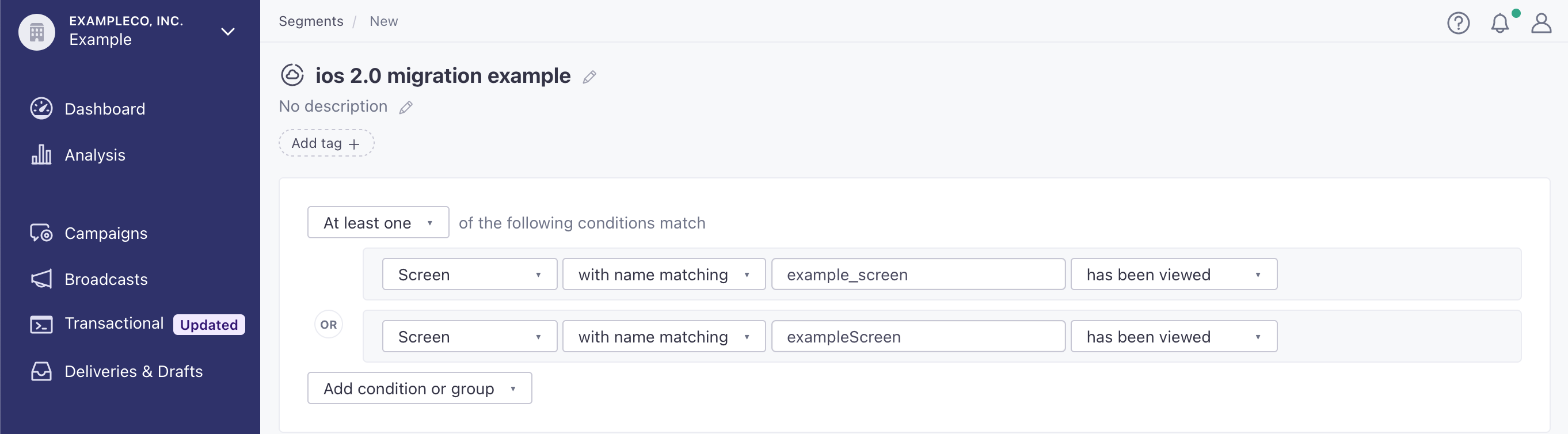
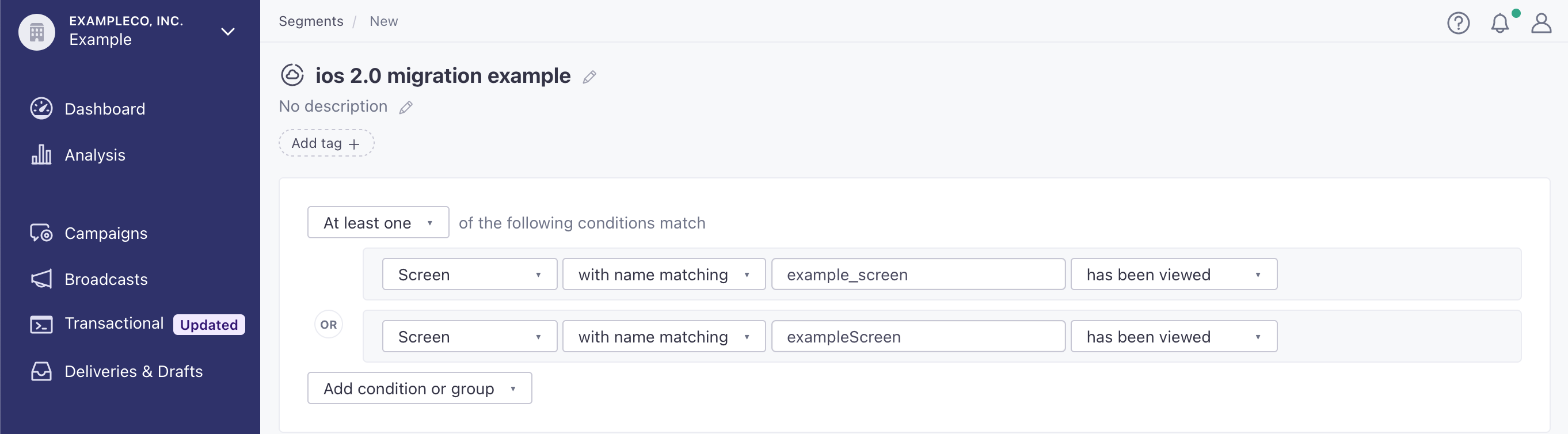
For Attributes: identify
, profileAttributes
, and deviceAttribute
calls
If your customer data was inappropriately snake-cased by the v1 SDK, you can set up a campaign to apply correctly formatted attributes in Customer.io so you don’t need to update your app! If you update your data this way, you may still need to update segments and other assets to use the correct data shape.
Create a segment of people possessing the affected, snake-cased attributes.
Create a campaign using this segment as a trigger.
Configure the first action to set correctly formatted attributes using the values from your previously-misshaped attributes. Use liquid to identify the attributes in question. Use a liquid or JS if statement to set an attribute value if it exists, otherwise your campaign may experience errors.
{% if customer.snake_case %}{{customer.snake_case}}{% endif %}
Configure the second Create or Update Person action to remove the bugged, snake-case attributes from your audience.
Make sure that your segmentsA group of people who match a series of conditions. People enter and exit the segment automatically when they match or stop matching conditions., filters, and other items that might be based on people’s attributes or device attributes are all set to use your preferred format.
Option 2: Use snake-cased formats in your app
// Call the Customer.io SDK and provide custom attributes like this:
CustomerIO.identify("dana@example.com", {"first_name": "Dana"})
// Consider sending duplicate data with snake_case
CustomerIO.identify("dana@example.com", {
"firstName": "Dana", // Attribute used with v1 of the SDK that got converted to snake_case. Keeping it here as the bug has been fixed.
"first_name": "Dana" // Adding this duplicate attribute for backwards compatibility with customers using old versions of your app.
})
Then, after you have determined that all of your app’s customers have updated their app to a version of your app no longer using v1 of the Customer.io SDK, you can remove this duplication:
CustomerIO.identify("dana@example.com", {
"firstName": "Dana" // We can remove the snake_case attribute and go back to just camelCase!
})